Reverse Geocoding in React Native with Kotlin/Java without Google Maps API
Steps
- Create two files ReverseGeocodingPackage.java and ReverseGeocodingModule.java under your main android project.
- Add below code in ReverseGeocodingPackage.java
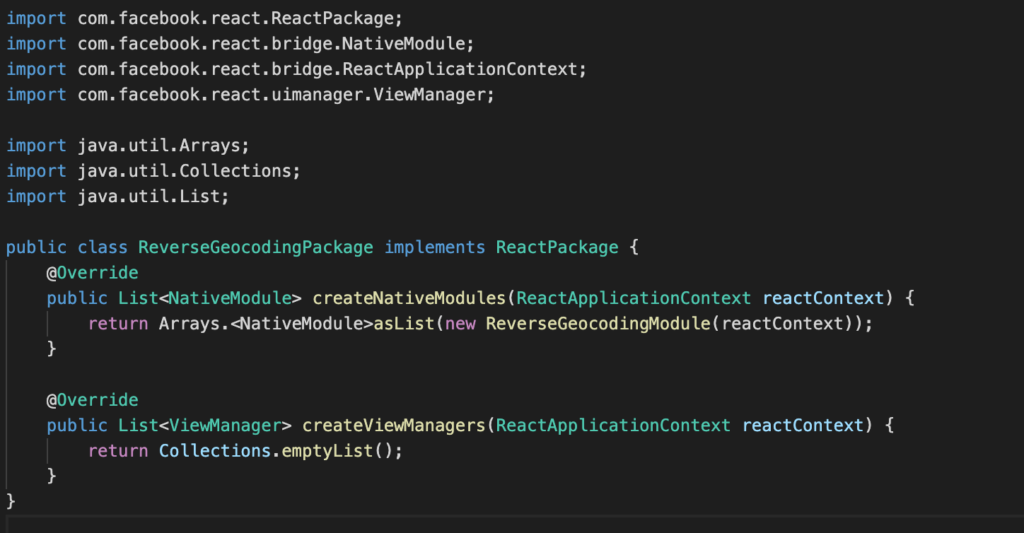
- Add below code in ReverseGeocodingModule.java
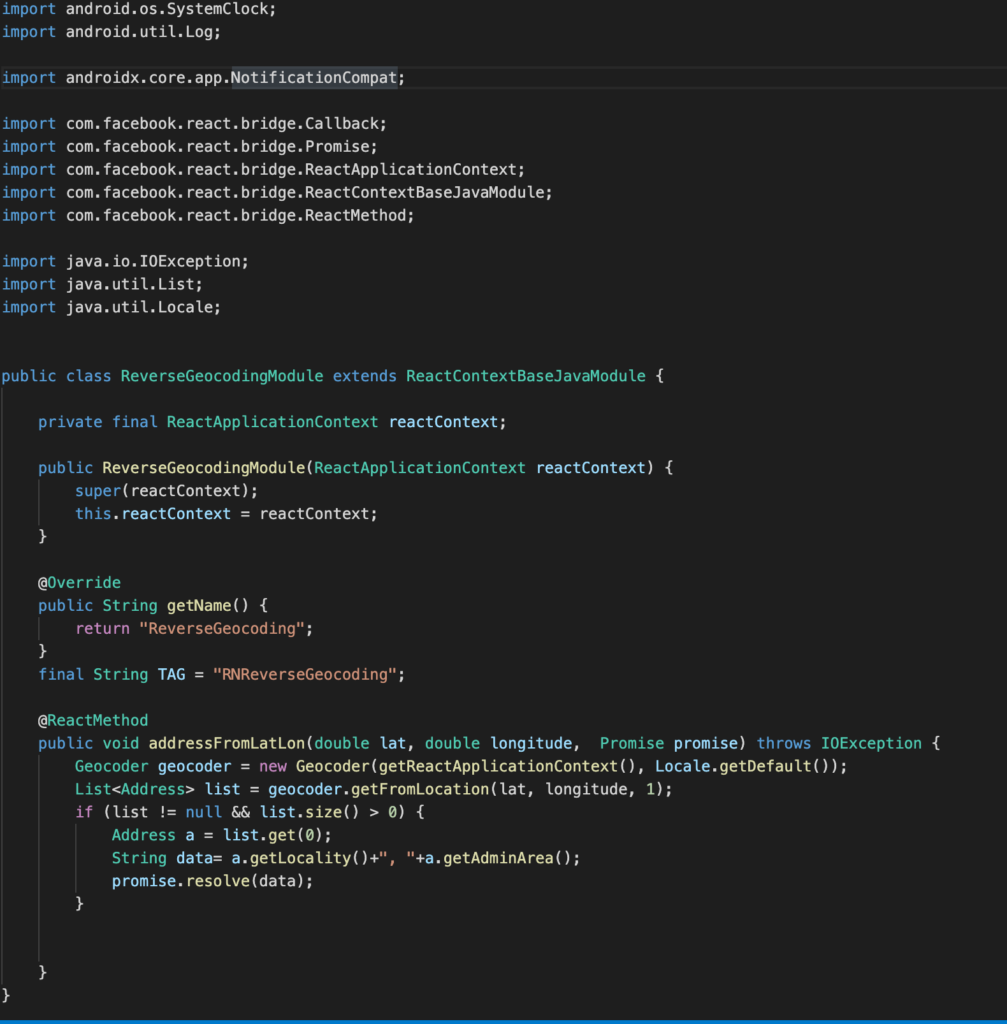
- Now, in the React- Native project you can call the above native code by importing NativeModules package.
- To fetch latitude and longitude, we have used ‘react-native-geolocation-service’.
import Geolocation from 'react-native-geolocation-service';
import {
NativeModules
} from 'react-native';
import ReverseGeocoding from '../../../ReverseGeocoding';
async userGeolocation() {
console.log("userGeolocation")
Geolocation.getCurrentPosition(async (info) =>
{
let latitude = info.coords.latitude;
let longitude = info.coords.longitude;
let timestamp = info.timestamp;
console.log("timestamp: " + timestamp)
this.setState({
timestamp
})
try {
if (Platform.OS === 'ios')
{
let data = await ReverseGeocodingNative.addressFromLatLon(latitude, longitude)
console.log("latLong: " + JSON.stringify(data))
console.log(data.City + ", " + data.State)
let location = data.City + ", " + data.State;
this.setState({
location
})
} else
{
let data = await ReverseGeocoding.addressFromLatLon(latitude, longitude)
console.log("latLong: " + JSON.stringify(data))
let location = data
this.setState({
location
})
}
return
} catch (e)
{
console.log("latLOng error: " + e)
return false
}
}
);
}